JS Tutorial
JS Version
JS Objects
JS Function
JS Classes
JS Async
JS HTML DOM
JS Browser BOM
JS Web API
JS AJAX
JS JSON
JS vs JQUERY
JS Graphics
JS Asynchronous
JavaScript Promise Object
A JavaScript Promise object consists of both the producing code and calls to the consuming code:
Promise Syntax
let myPromise = new Promise(function(myResolve, myReject) {
// “Producing Code” (May take some time)
myResolve(); // when successful
myReject(); // when error
});
// “Consuming Code” (Must wait for a fulfilled Promise)
myPromise.then(
function(value) { /* code if successful */ },
function(error) { /* code if some error */ }
);
Promise Object Properties
A JavaScript Promise object can be:
- Pending
- Fullfilled
- Rejected
The Promise object supports two properties: state and result.
When a Promise object is “pending” (working), the result is undefined.
When a Promise object is “fulfilled”, the result is a value.
When a Promise object is “rejected”, the result is an error object.
Promise How To
Below is an example that makes use a Promise:
myPromise.then(
function(value) { /* code if successful */ },
function(error) { /* code if some error */ }
);
Promise.then() takes passes two arguments, a callback for success and another for failure.
Both are optional, you can add a callback for success or failure only.
Example
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Promise</h2>
<p id=”demo”></p>
<script>
function myDisplayer(some) { document.getElementById(“demo”).innerHTML = some;
}
let myPromise = new Promise(function(myResolve, myReject) {
let x = 0;
// some code (try to change x to 5)
if (x == 0) {
myResolve(“OK”);
} else {
myReject(“Error”);
}
});
myPromise.then(
function(value) {myDisplayer(value);},
function(error) {myDisplayer(error);}
);
</script>
</body>
</html>
Output
JavaScript Promise
OK
JavaScript Promise Examples
Waiting for a Timeout
Example (Using Callback)
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript SetTimeout()</h2>
<p>Wait 3 seconds (3000 milliseconds) for this page to change.</p>
<h2 id=”demo”></h2>
<script>
setTimeout(function() { myFunction(“Hi !!!”); }, 3000);
function myFunction(value) {
document.getElementById(“demo”).innerHTML = value;
}
</script>
</body>
</html>
Output
JavaScript SetTimeout()
Wait 3 seconds (3000 milliseconds) for this page to change.
Example (Using Promise)
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Promise</h2>
<p>Wait 3 seconds (3000 milliseconds) for this page to change.</p>
<h2 id=”demo”></h2>
<script>
const myPromise = new Promise(function(myResolve, myReject) {
setTimeout(function(){ myResolve(“Nice !!”); }, 3000);
});
myPromise.then(function(value) {
document.getElementById(“demo”).innerHTML = value;
});
</script>
</body>
</html>
Output
JavaScript Promise
Wait 3 seconds (3000 milliseconds) for this page to change.
Waiting for a file
Example (using Callback)
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Callbacks</h2>
<p id=”demo”></p>
<script>
function myDisplayer(some) {
document.getElementById(“demo”).innerHTML = some;
}
function getFile(myCallback) {
let req = new XMLHttpRequest();
req.open(‘GET’, “mycar.html”);
req.onload = function() {
if (req.status == 200) {
myCallback(this.responseText);
} else {
myCallback(“Error: ” + req.status);
}
}
req.send();
}
getFile(myDisplayer);
</script>
</body>
</html>
Output
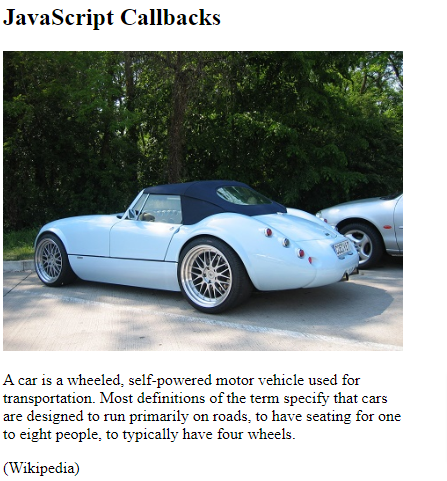
Example (using Promise)
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Callbacks</h2>
<p id=”demo”></p>
<script>
function myDisplayer(some) {
document.getElementById(“demo”).innerHTML = some;
}
function getFile(myCallback) {
let req = new XMLHttpRequest();
req.open(‘GET’, “mycar.html”);
req.onload = function() {
if (req.status == 200) {
myCallback(this.responseText);
} else {
myCallback(“Error: ” + req.status);
}
}
req.send();
}
getFile(myDisplayer);
</script>
</body>
</html>
Output
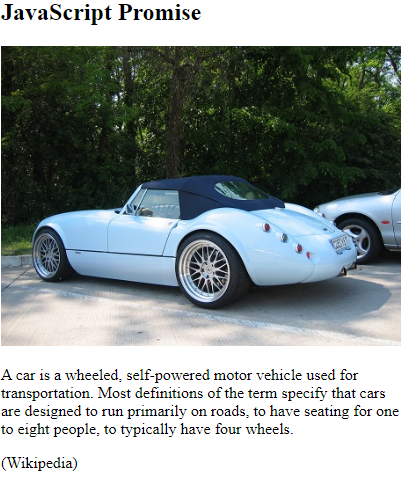