JS Tutorial
JS Version
JS Objects
JS Function
JS Classes
JS Async
JS HTML DOM
JS Browser BOM
JS Web API
JS AJAX
JS JSON
JS vs JQUERY
JS Graphics
JS Asynchronous
Async Syntax
Adding the keyword async before a function makes the function returns a promise.
async function myFunction() {
return “Hello”;
}
Is the same as:
function myFunction() {
return Promise.resolve(“Hello”);
}
Here is how to use the Promise:
myFunction().then(
function(value) { /* code if successful */ },
function(error) { /* code if some error */ }
);
Example
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript async / await</h2>
<p id=”demo”></p>
<script>
function myDisplayer(some) {
document.getElementById(“demo”).innerHTML = some;
}
async function myFunction() {return “Hello”;}
myFunction().then(
function(value) {myDisplayer(value);},
function(error) {myDisplayer(error);}
);</script>
</body>
</html>
Output
JavaScript async / await
If you expect a normal value (a normal response, not an error).
Example
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript async / await</h2>
<p id=”demo”></p>
<script>
function myDisplayer(some) {
document.getElementById(“demo”).innerHTML = some;
}
async function myFunction() {return “Hello”;}
myFunction().then(
function(value) {myDisplayer(value);}
);
</script>
</body>
</html>
Output
JavaScript async / await
Hello
Await Syntax
The keyword awaits before a function makes the function wait for a promise:
let value = await promise;
The await keyword can only be used within an async function.
Example
Let’s go slowly and learn how to use it.
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript async / await</h2>
<h1 id=”demo”></h1>
<script>
async function myDisplay() {
let myPromise = new Promise(function(resolve, reject) {
resolve(“Nice !!”);
});
document.getElementById(“demo”).innerHTML = await myPromise;
}
myDisplay();
</script>
</body>
</html>
Output
JavaScript async / await
Nice !!
Example without reject
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript async / await</h2>
<h1 id=”demo”></h1>
<script>
async function myDisplay() {
let myPromise = new Promise(function(resolve) {
resolve(“Nice !!”);
});
document.getElementById(“demo”).innerHTML = await myPromise;
}
myDisplay();
</script>
</body>
</html>
Output
JavaScript async / await
Nice !!
Waiting for a Timeout
Example
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript async / await</h2>
<p>Wait 3 seconds (3000 milliseconds) for this page to change.</p>
<h2 id=”demo”></h2>
<script>
async function myDisplay() {
let myPromise = new Promise(function(resolve) {
setTimeout(function() {resolve(“Yupp !!”);}, 3000);
});
document.getElementById(“demo”).innerHTML = await myPromise;
}
myDisplay();
</script>
</body>
</html>
Output
JavaScript async / await
Wait 3 seconds (3000 milliseconds) for this page to change.
Waiting for a File
Example
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript async / await</h2>
<p id=”demo”></p>
<script>
async function getFile() {
let myPromise = new Promise(function(resolve) {
let req = new XMLHttpRequest();
req.open(‘GET’, “mycar.html”);
req.onload = function() {
if (req.status == 200) {
resolve(req.response);
} else {
resolve(“File not Found”);
}
};
req.send();
});
document.getElementById(“demo”).innerHTML = await myPromise;
}
getFile();
</script>
</body>
</html>
Output
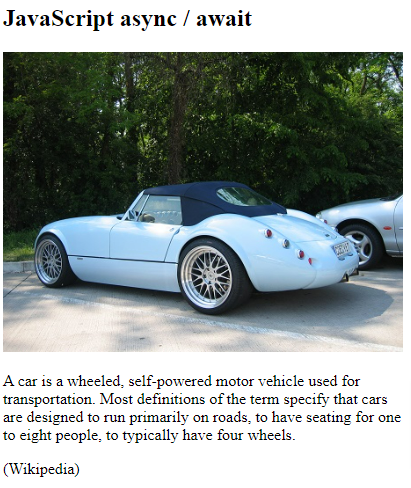