HTML Tutorial
Menu
HTML Forms
Menu
HTML Graphics
Menu
HTML Media
Menu
HTML API
Menu
HTML Canvas Graphics
The HTML <canvas> element helps to draw graphics on a web page.
What is HTML Canvas?
- The HTML <canvas> element draws graphics, on the fly, through JavaScript.
- The <canvas> element acts as a container only for graphics. JavaScript must be used to actually draw the graphics.
- Canvas provides several methods for drawing paths, boxes, circles, text, and adding images.
Canvas Examples
Add a JavaScript.
After the rectangular canvas area is created, JavaScript should be added to do the drawing.
Here are some examples:
Draw a Line
Output:

Draw A Circle
Output:
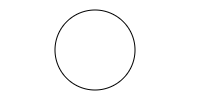
Draw A Text
Output:
Hello World
Draw a linear Gradient
Output:

Draw Circular Gradient
Output:
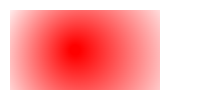