This tutorial shows you Laravel 9 crud with image upload. Let’s see below the example Laravel 9 crud example with image upload. You will get the basic crud with image upload stuff using controller, model, route, bootstrap 5, and blade.
.env DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=here your database name(blog) DB_USERNAME=here database username(root) DB_PASSWORD=here database password(root)
Step 1: Install laravel 9
If you haven’t created the Laravel app, then you can use the below command:
composer create-project laravel/laravel example-app
Step 2: Database Configuration
Let’s make a database configuration for the example database name, username, password, etc for our crud application of Laravel 9. Open the .env file and fill in all details like as below
.env DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=here your database name(blog) DB_USERNAME=here database username(root) DB_PASSWORD=here database password(root)
Step 3: Create a Migration
Let’s create a crud application for product and migration for the “products” table using Laravel 9 PHP artisan command, so use the below command:
php artisan make:migration create_products_table --create=products
After using this command, you will find this one file in the following path “database/migrations” use the below code in your migration file to create a products table.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('products', function (Blueprint $table) { $table->id(); $table->string('name'); $table->text('detail'); $table->string('image'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('products'); } };
Run this migration by the following command:
php artisan migrate
Step 4: Add Controller and Model
Create a new controller as ProductController. in this controller we’ll be writing logic to store images, which will be stored in the “images” folder of the public folder. Run the below command and create a new controller. Below controller for creating a resource controller.
php artisan make:controller ProductController --resource --model=Product
After the below command, you will find a new file in this path:
app/Http/Controllers/ProductController.php
In this controller will create seven methods by default as bellow methods:
- index()
- create()
- store()
- show()
- edit()
- update()
- destroy()
Copy the below code and put it in the ProductController.php file.
app/Http/Controllers/ProductController.php
<?php namespace App\Http\Controllers; use App\Models\Product; use Illuminate\Http\Request; class ProductController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { $products = Product::latest()->paginate(5); return view('products.index',compact('products')) ->with('i', (request()->input('page', 1) - 1) * 5); } /** * Show the form for creating a new resource. * * @return \Illuminate\Http\Response */ public function create() { return view('products.create'); } /** * Store a newly created resource in storage. * * @param \Illuminate\Http\Request $request * @return \Illuminate\Http\Response */ public function store(Request $request) { $request->validate([ 'name' => 'required', 'detail' => 'required', 'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048', ]); $input = $request->all(); if ($image = $request->file('image')) { $destinationPath = 'images/'; $profileImage = date('YmdHis') . "." . $image->getClientOriginalExtension(); $image->move($destinationPath, $profileImage); $input['image'] = "$profileImage"; } Product::create($input); return redirect()->route('products.index') ->with('success','Product created successfully.'); } /** * Display the specified resource. * * @param \App\Product $product * @return \Illuminate\Http\Response */ public function show(Product $product) { return view('products.show',compact('product')); } /** * Show the form for editing the specified resource. * * @param \App\Product $product * @return \Illuminate\Http\Response */ public function edit(Product $product) { return view('products.edit',compact('product')); } /** * Update the specified resource in storage. * * @param \Illuminate\Http\Request $request * @param \App\Product $product * @return \Illuminate\Http\Response */ public function update(Request $request, Product $product) { $request->validate([ 'name' => 'required', 'detail' => 'required' ]); $input = $request->all(); if ($image = $request->file('image')) { $destinationPath = 'images/'; $profileImage = date('YmdHis') . "." . $image->getClientOriginalExtension(); $image->move($destinationPath, $profileImage); $input['image'] = "$profileImage"; }else{ unset($input['image']); } $product->update($input); return redirect()->route('products.index') ->with('success','Product updated successfully'); } /** * Remove the specified resource from storage. * * @param \App\Product $product * @return \Illuminate\Http\Response */ public function destroy(Product $product) { $product->delete(); return redirect()->route('products.index') ->with('success','Product deleted successfully'); } }
Now, after doing this run the below command, you will be finding “app/Models/Product.php” and put below content in the Product.php file:
app/Models/Product.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Product extends Model { use HasFactory; protected $fillable = [ 'name', 'detail', 'image' ]; }
Step 5: Add Resource Route
Here, we need to add a resource route for the product crud application. so open your “routes/web.php” file and add the following route.
routes/web.php
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\ProductController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::resource('products', ProductController::class);
Step 6: Add Blade Files
Let’s create just blade files. Mainly, we will be focusing on creating layout files and after that, we will create a new folder “products” and then create blade files of the crud app. Finally, we will create following below blade file:
- layout.blade.php
- index.blade.php
- create.blade.php
- edit.blade.php
- show.blade.php
Create the following file and put the below code.
resources/views/products/layout.blade.php
<!DOCTYPE html> <html> <head> <title>Laravel 9 CRUD with Image Upload Application</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"> </head> <body> <div class="container"> @yield('content') </div> </body> </html>
resources/views/products/index.blade.php
@extends('products.layout') @section('content') <div class="row"> <div class="col-lg-12 margin-tb"> <div class="pull-left"> <h2>Laravel 9 CRUD with Image Upload Example from scratch</h2> </div> <div class="pull-right"> <a class="btn btn-success" href="{{ route('products.create') }}"> Create New Product</a> </div> </div> </div> @if ($message = Session::get('success')) <div class="alert alert-success"> <p>{{ $message }}</p> </div> @endif <table class="table table-bordered"> <tr> <th>No</th> <th>Image</th> <th>Name</th> <th>Details</th> <th width="280px">Action</th> </tr> @foreach ($products as $product) <tr> <td>{{ ++$i }}</td> <td><img src="/images/{{ $product->image }}" width="100px"></td> <td>{{ $product->name }}</td> <td>{{ $product->detail }}</td> <td> <form action="{{ route('products.destroy',$product->id) }}" method="POST"> <a class="btn btn-info" href="{{ route('products.show',$product->id) }}">Show</a> <a class="btn btn-primary" href="{{ route('products.edit',$product->id) }}">Edit</a> @csrf @method('DELETE') <button type="submit" class="btn btn-danger">Delete</button> </form> </td> </tr> @endforeach </table> {!! $products->links() !!} @endsection
resources/views/products/create.blade.php
@extends('products.layout') @section('content') <div class="row"> <div class="col-lg-12 margin-tb"> <div class="pull-left"> <h2>Add New Product</h2> </div> <div class="pull-right"> <a class="btn btn-primary" href="{{ route('products.index') }}"> Back</a> </div> </div> </div> @if ($errors->any()) <div class="alert alert-danger"> <strong>Whoops!</strong> There were some problems with your input.<br><br> <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif <form action="{{ route('products.store') }}" method="POST" enctype="multipart/form-data"> @csrf <div class="row"> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Name:</strong> <input type="text" name="name" class="form-control" placeholder="Name"> </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Detail:</strong> <textarea class="form-control" style="height:150px" name="detail" placeholder="Detail"></textarea> </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Image:</strong> <input type="file" name="image" class="form-control" placeholder="image"> </div> </div> <div class="col-xs-12 col-sm-12 col-md-12 text-center"> <button type="submit" class="btn btn-primary">Submit</button> </div> </div> </form> @endsection
resources/views/products/edit.blade.php
@extends('products.layout') @section('content') <div class="row"> <div class="col-lg-12 margin-tb"> <div class="pull-left"> <h2>Edit Product</h2> </div> <div class="pull-right"> <a class="btn btn-primary" href="{{ route('products.index') }}"> Back</a> </div> </div> </div> @if ($errors->any()) <div class="alert alert-danger"> <strong>Whoops!</strong> There were some problems with your input.<br><br> <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif <form action="{{ route('products.update',$product->id) }}" method="POST" enctype="multipart/form-data"> @csrf @method('PUT') <div class="row"> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Name:</strong> <input type="text" name="name" value="{{ $product->name }}" class="form-control" placeholder="Name"> </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Detail:</strong> <textarea class="form-control" style="height:150px" name="detail" placeholder="Detail">{{ $product->detail }}</textarea> </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Image:</strong> <input type="file" name="image" class="form-control" placeholder="image"> <img src="/images/{{ $product->image }}" width="300px"> </div> </div> <div class="col-xs-12 col-sm-12 col-md-12 text-center"> <button type="submit" class="btn btn-primary">Submit</button> </div> </div> </form> @endsection
resources/views/products/show.blade.php
@extends('products.layout') @section('content') <div class="row"> <div class="col-lg-12 margin-tb"> <div class="pull-left"> <h2> Show Product</h2> </div> <div class="pull-right"> <a class="btn btn-primary" href="{{ route('products.index') }}"> Back</a> </div> </div> </div> <div class="row"> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Name:</strong> {{ $product->name }} </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Details:</strong> {{ $product->detail }} </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Image:</strong> <img src="/images/{{ $product->image }}" width="500px"> </div> </div> </div> @endsection
Run Laravel App
Type the given below command and hit enter to run the Laravel app:
php artisan serve
Now, navigate our web browser, type the given URL and view the app output:
Make sure, you have created an “images” folder in the public directory.
You will see the layout below:
List Page:
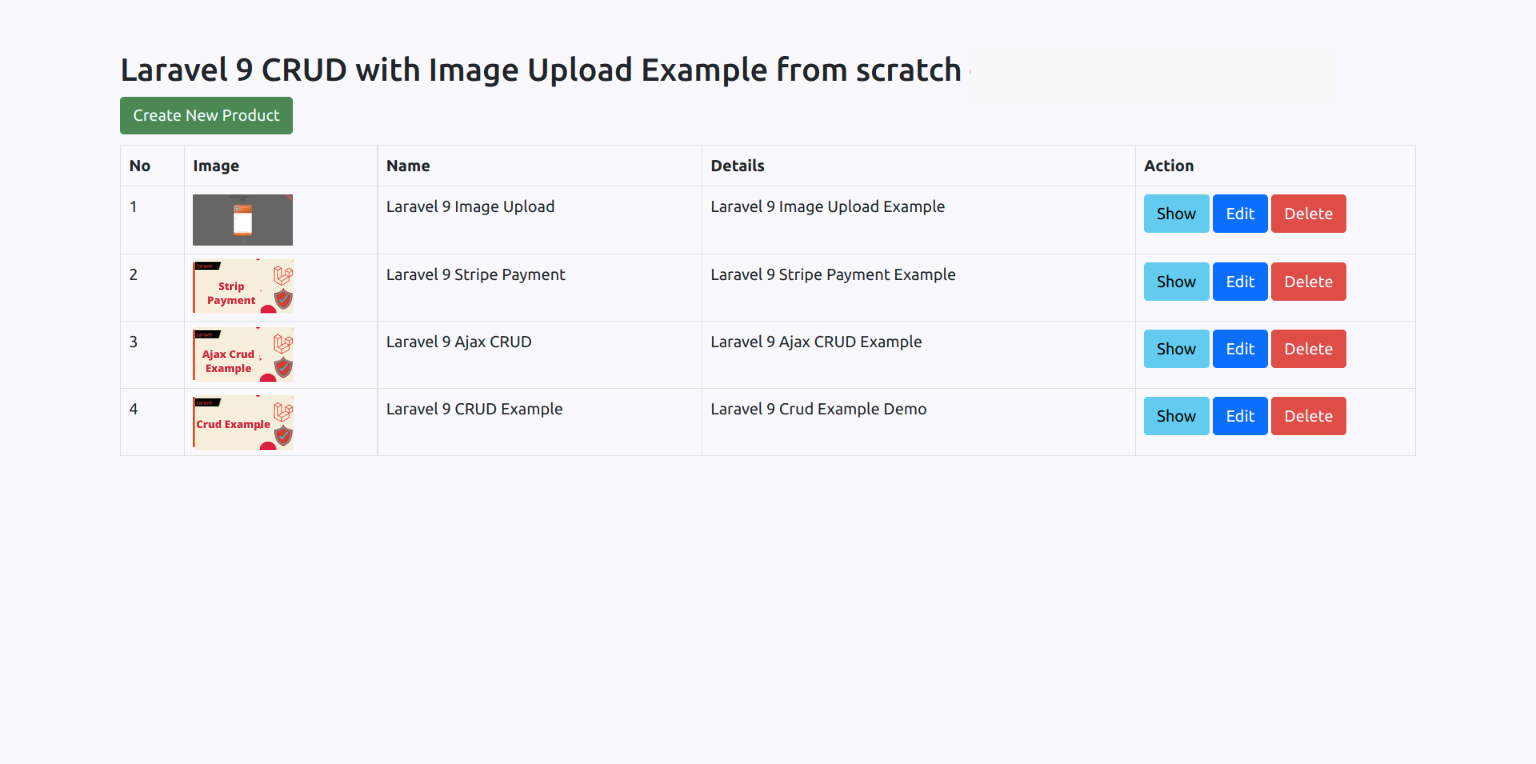